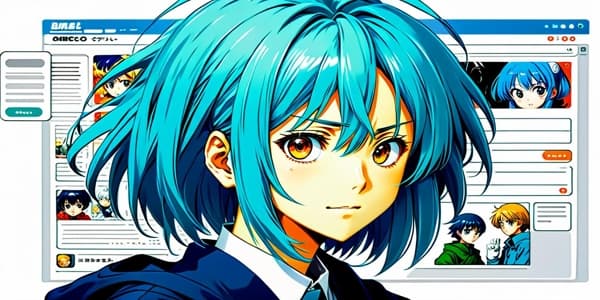
Integrate Waline with Next.js
Waline: A Lightweight and Modern Comment System
Waline is an open-source comment system designed to be lightweight, fast, and easy to integrate. It is particularly suitable for statically generated blogs and websites, which is also possible with Next.js.
Unlike other comment systems that can slow down your pages, Waline uses a serverless database architecture, ensuring optimal performance.
Even though at one point I wanted to create my own comment system with the Prisma ORM, this is ultimately the solution I chose for my own website, the one you are visiting right now!
The Advantages of Waline
- Lightweight and fast: Waline is designed to minimize the impact on your site's performance.
- Open source: you can customize Waline according to your needs and contribute to its development.
- Serverless: Uses lambda functions to manage comments, meaning you don't have to manage dedicated servers.
- Markdown support: Allows users to format their comments with Markdown.
- Customizable: You can easily customize the appearance and behavior of Waline to suit your site.
Integrate Waline into your Next.js project
In this example, we will also use Typescript and Tailwind.css.
Here is a step-by-step guide to integrate Waline into your Next.js project.
If needed, here are the official links for Waline:
- Make sure you have a Next.js project set up. If you don't, you can create a new project using create-next-app:
npx create-next-app my-nextjs-blog
cd my-nextjs-blog
- Install Waline
Waline provides a simple JavaScript interface that you can add to your project. First you need to install the Waline package:
npm, pnpm or yarn:
npm install @waline/client
pnpm i @waline/client
yarn add @waline/client
- Configure Backend:
Waline requires a backend and a database to store comments.
Full instructions for deploying the backend are available in the Waline documentation, you can follow the official guide.
For more options regarding your database, take a look at this page
For more options regarding the actual deployment of the backend, look under "Deploy" on their documentation page.
- Add Waline to your Next.js Project:
Create a new component to integrate Waline. Add a WalineComments.tsx file in your "components" folder:
// components/WalineComments.tsx
'use client'
import { useEffect } from 'react';
import Waline from '@waline/client';
interface WalineCommentsProps {
className?: string;
}
const WalineComments: React.FC<WalineCommentsProps> = ({ className }) => {
useEffect(() => {
init({
el: '#waline',
lang: 'en', // change depending on your language
reaction: true,
serverURL: 'https://your-waline-server.vercel.app', // change the url of your server there
emoji: [
'https://cdn.jsdelivr.net/gh/walinejs/emojis@1.0.0/weibo',
'https://cdn.jsdelivr.net/gh/walinejs/emojis@1.0.0/alus',
],
requiredMeta: ['nick'],
})});
return <div id="waline" className={`${className}`} />;
};
export default WalineComments;
- Customize the css:
Create a walinecustom.css file, copy-paste the following code, and adapt it according to your tastes/themes or needs.
#waline {
--waline-theme-color: #677DE1;
--waline-active-color: #7790ff;
/* Regular color */
--waline-white: #fff;
--waline-light-grey: #b3b3b3;
--waline-dark-grey: #383838;
/* Information */
--waline-info-font-size: 0.625em;
/* Layout Color */
--waline-color: #c47676;
--waline-bg-color: #fff;
--waline-bg-color-light: #f8f8f8;
--waline-bg-color-hover: #f0f0f0;
--waline-border-color: #ddd;
--waline-disable-bg-color: #f8f8f8;
--waline-disable-color: #bbb;
--waline-code-bg-color: #282c34;
}
#waline .wl-comment {
@apply gap-x-4;
}
#waline .wl-panel {
margin: 0;
}
#waline .wl-editor {
width: calc(100% - 2.4rem);
}
Import this .css file in your main tailwind.css file:
@import 'walinecustom.css';
- Use Waline component in your pages:
Use this component in pages where you want to display comments. For example, in a blog post.tsx page:
import { FC } from 'react';
import WalineComments from '@/components/WalineComments';
interface PostProps {
params: {
slug: string;
};
}
const Post: FC<PostProps> = ({ params }) => {
const { slug } = params;
// Simulate a data recovery of your post here
const post = { title: 'My First Post', content: 'This is the content of my first post.' };
return (
<div className='flex flex-col items-center justify-center'>
<h1>{post.title}</h1>
<p>{post.content}</p>
<WalineComments className=''/>
</div>
);
};
export default Post;
- Deploy your project:
After configuring Waline and updating your pages, deploy your Next.js project to your preferred hosting platform. Make sure your Waline backend is also deployed and configured properly.
Add a view counter
Create an icon.tsx component for the view counter:
// components/icon.tsx
import { SVGProps } from 'react'
export function Eye(svgProps: SVGProps<SVGSVGElement>) {
return (
<svg
{...svgProps}
xmlns="http://www.w3.org/2000/svg"
height="18"
width="18"
fill="currentColor"
viewBox="0 0 576 512"
>
<path d="M288 32c-80.8 0-145.5 36.8-192.6 80.6C48.6 156 17.3 208 2.5 243.7c-3.3 7.9-3.3 16.7 0 24.6C17.3 304 48.6 356 95.4 399.4C142.5 443.2 207.2 480 288 480s145.5-36.8 192.6-80.6c46.8-43.5 78.1-95.4 93-131.1c3.3-7.9 3.3-16.7 0-24.6c-14.9-35.7-46.2-87.7-93-131.1C433.5 68.8 368.8 32 288 32zM144 256a144 144 0 1 1 288 0 144 144 0 1 1 -288 0zm144-64c0 35.3-28.7 64-64 64c-7.1 0-13.9-1.2-20.3-3.3c-5.5-1.8-11.9 1.6-11.7 7.4c.3 6.9 1.3 13.8 3.2 20.7c13.7 51.2 66.4 81.6 117.6 67.9s81.6-66.4 67.9-117.6c-11.1-41.5-47.8-69.4-88.6-71.1c-5.8-.2-9.2 6.1-7.4 11.7c2.1 6.4 3.3 13.2 3.3 20.3z" />
</svg>
)
}
Create a new component PageViews.tsx, then add the following code:
// components/PageViews.tsx
'use client';
import React, { useEffect } from 'react';
import { useParams } from 'next/navigation';
import { pageviewCount } from '@waline/client';
import { Eye } from './icons';
interface PageviewProps {
path: string;
className?: string;
}
export const Pageview: React.FC<PageviewProps> = ({ path, className }) => {
const handlePageviewDataFetch = async () => {
await pageviewCount({
serverURL: 'https://your-waline-server.vercel.app', // change the URL of your server here
path: path,
});
};
useEffect(() => {
handlePageviewDataFetch();
}, [path]);
return (
<div className={`${className} flex flex-row items-center`}>
<Eye className={'mr-2'} />
<p className="mr-2">View</p>
<span className="waline-pageview-count mr-2" data-path={path} />
<p>times</p>
</div>
);
};
Modify your test page like this:
import { FC } from 'react';
import WalineComments from '@/components/WalineComments';
import PageViews from '@/components/PageViews';
interface PostProps {
params: {
slug: string;
};
}
const Post: FC<PostProps> = ({ params }) => {
const { slug } = params;
// Simulate a data recovery of your post here
const post = { title: 'My First Post', content: 'This is the content of my first post.' };
return (
<div className='flex flex-col items-center justify-center'>
<h1>{post.title}</h1>
<PageViews className=''/>
<p>{post.content}</p>
<WalineComments className=''/>
</div>
);
};
export default Post;
Note: you will probably see some errors in local development, but this is normal, do not take it into account. If you have configured everything properly, there will be no problem in production.
Of course, do not forget to style these components, and you will be ready to share your articles with your audience. By adapting the provided code, an integration with i18n (supported by the Waline library) is also possible! Not all languages are available, but since Waline is open-source, you can request an update by providing the translations.
Conclusion
Waline is a modern, innovative and lightweight commenting solution that integrates perfectly with Next.js. By following these steps, you can easily add a powerful and customizable commenting system to your blog or website. For more details and advanced configurations, check out their official documentation!